1. Collision
1. 충돌
Have you ever been in a car accident? What did you collide with? Just like with cars, PhysicBody objects can come in contact.
교통 사고를 당하신 적이 있습니까? 무엇과 충돌 했습니까? 자동차와 마찬가지로 물리적 인 물체도 접촉 할 수 있습니다.
Collisions are what happens when PhysicBody objects come in contact with each other.
충돌은 물리적 인 물체가 서로 접촉 할 때 일어나는 일입니다.
When a collision takes place it can be ignored or it can trigger events to be fired.
충돌이 발생하면 무시하거나 이벤트를 발생시킬 수 있습니다.
2. Filtering Collisions
3. 충돌 필터링
Collision filtering allows you to enable or prevent collisions between shapes.
충돌 필터링을 사용하면 모양 간의 충돌을 활성화하거나 방지 할 수 있습니다.
This physics engine supports collision filtering using category and group bitmasks.
이 물리 엔진은 카테고리 및 그룹 비트 마스크를 사용한 충돌 필터링을 지원합니다.
There are 32 supported collision categories.
지원되는 충돌 범주는 32 개입니다.
For each shape you can specify which category it belongs to.
각 도형에 대해 어떤 카테고리에 속하는지 지정할 수 있습니다.
You can also specify what other categories this shape can collide with.
이 모양이 충돌 할 수있는 다른 범주를 지정할 수도 있습니다.
This is done with masking bits.
이것은 마스킹 비트로 수행됩니다.
For example:
auto visibleSize = Director::getInstance()->getVisibleSize();
s_centre = Vec2(visibleSize.width/2, visibleSize.height/2);
auto sprite1 = addSpriteAtPosition(Vec2(s_centre.x - 150,s_centre.y));
sprite1->getPhysicsBody()->setCategoryBitmask(0x02); // 0010
sprite1->getPhysicsBody()->setCollisionBitmask(0x01); // 0001
sprite1 = addSpriteAtPosition(Vec2(s_centre.x - 150,s_centre.y + 100));
sprite1->getPhysicsBody()->setCategoryBitmask(0x02); // 0010
sprite1->getPhysicsBody()->setCollisionBitmask(0x01); // 0001
auto sprite2 = addSpriteAtPosition(Vec2(s_centre.x + 150,s_centre.y),1);
sprite2->getPhysicsBody()->setCategoryBitmask(0x01); // 0001
sprite2->getPhysicsBody()->setCollisionBitmask(0x02); // 0010
auto sprite3 = addSpriteAtPosition(Vec2(s_centre.x + 150,s_centre.y + 100),2);
sprite3->getPhysicsBody()->setCategoryBitmask(0x03); // 0011
sprite3->getPhysicsBody()->setCollisionBitmask(0x03); // 0011
You can check for collisions by checking and comparing category and collision bitmasks like:
다음과 같은 카테고리 및 충돌 비트 마스크를 확인하고 비교하여 충돌을 확인할 수 있습니다.
if ((shapeA->getCategoryBitmask() & shapeB->getCollisionBitmask()) == 0
|| (shapeB->getCategoryBitmask() & shapeA->getCollisionBitmask()) == 0)
{
// shapes can't collide
ret = false;
}
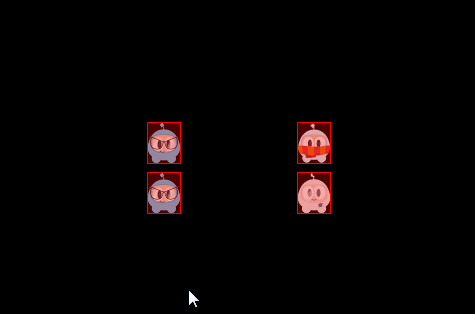
Collision groups let you specify an integral group index.
충돌 그룹을 사용하면 통합 그룹 인덱스를 지정할 수 있습니다.
You can have all shapes with the same group index always collide (positive index) or never collide (negative index and zero index).
동일한 그룹 인덱스를 가진 모든 셰이프가 항상 충돌 (양수 인덱스)하거나 절대 충돌 (음수 인덱스 및 0 인덱스)하도록 할 수 있습니다.
Collisions between shapes of different group indices are filtered according the category and mask bits.
서로 다른 그룹 인덱스의 모양 간의 충돌은 범주 및 마스크 비트에 따라 필터링됩니다.
In other words, group filtering has higher precedence than category filtering.
즉, 그룹 필터링은 범주 필터링보다 우선 순위가 높습니다.
3. Contacts/Joints
3. 접점 / 조인트
Recall from the terminology above that joints are how contact points are connected to each other.
위의 용어에서 관절은 접점이 서로 연결되는 방식이라는 것을 기억하십시오.
Yes, you can think of it just like joints on your own body.
예, 당신은 자신의 몸에있는 관절처럼 생각할 수 있습니다.
Each joint type has a definition that derives from PhysicsJoint.
각 관절 유형에는 물리 관절에서 파생 된 정의가 있습니다.
All joints are connected between two different bodies.
모든 관절은 서로 다른 두 몸체 사이에 연결됩니다.
One body may be static.
한 몸은 정적 일 수 있습니다.
You can prevent the attached bodies from colliding with each other by joint->setCollisionEnable(false).
joint-> setCollisionEnable (false)로 부착 된 바디가 서로 충돌하는 것을 방지 할 수 있습니다.
Many joint definitions require that you provide some geometric data.
많은 접합 정의에서는 일부 기하학적 데이터를 제공해야합니다.
Often a joint will be defined by anchor points.
종종 조인트는 앵커 포인트로 정의됩니다.
The rest of the joint definition data depends on the joint type.
나머지 조인트 정의 데이터는 조인트 유형에 따라 다릅니다.
-PhysicsJointFixed: A fixed joint fuses the two bodies together at a reference point.
고정 조인트는 기준점에서 두 본체를 결합합니다.
Fixed joints are useful for creating complex shapes that can be broken apart later.
고정 관절은 나중에 분리 할 수있는 복잡한 모양을 만드는 데 유용합니다.
-PhysicsJointLimit: A limit joint imposes a maximum distance between the two bodies, as if they were connected by a rope.
제한 조인트는 마치 로프로 연결된 것처럼 두 본체 사이에 최대 거리를 부과합니다.
-PhysicsJointPin: A pin joint allows the two bodies to independently rotate around the anchor point as if pinned together.
핀 조인트를 사용하면 두 본체가 마치 함께 고정 된 것처럼 앵커 포인트를 중심으로 독립적으로 회전 할 수 있습니다.
-PhysicsJointDistance: Set the fixed distance with two bodies
두 몸체로 고정 거리 설정
-PhysicsJointSpring: Connecting two physics bodies together with a spring
두 개의 물리 몸체를 스프링으로 연결
-PhysicsJointGroove: Attach body a to a line, and attach body b to a dot
본문 a를 선에 연결하고 본문 b를 점에 연결
-PhysicsJointRotarySpring: Likes a spring joint, but works with rotary
스프링 조인트를 좋아하지만 로터리와 함께 작동합니다.
-PhysicsJointRotaryLimit: Likes a limit joint, but works with rotary
리미트 조인트를 좋아하지만 로터리와 함께 작동합니다.
-PhysicsJointRatchet: Works like a socket wrench
소켓 렌치처럼 작동
-PhysicsJointGear: Keeps the angular velocity ratio of a pair of bodies constant
한 쌍의 몸체의 각속도 비율을 일정하게 유지합니다.
-PhysicsJointMotor: Keeps the relative angular velocity of a pair of bodies constant
한 쌍의 몸체의 상대 각속도를 일정하게 유지합니다.
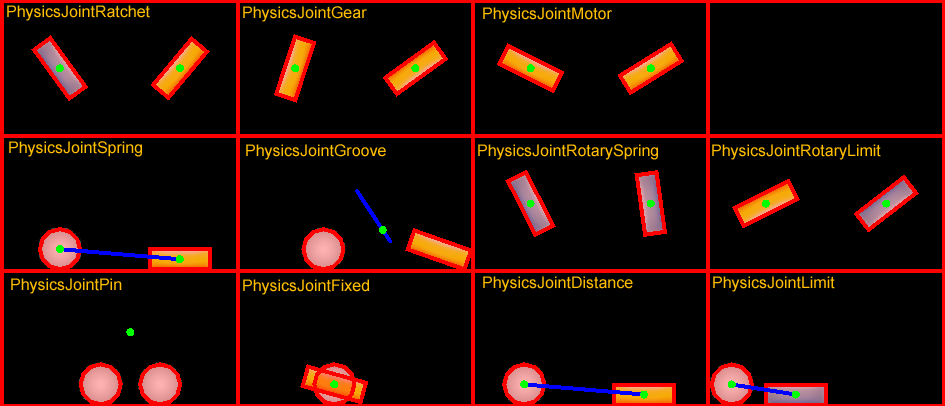
4. Collision detection
4. 충돌 감지
Contacts are objects created by the physics engine to manage the collision between two shapes.
접촉은 두 모양 간의 충돌을 관리하기 위해 물리 엔진에 의해 생성 된 객체입니다.
Contact objects are not created by the user, they are created automatically.
연락처 개체는 사용자가 만들지 않고 자동으로 만들어집니다.
There are a few terms associated with contacts.
연락처와 관련된 몇 가지 용어가 있습니다.
-contact point: A contact point is a point where two shapes touch.
접점은 두 도형이 만나는 지점입니다.
-contact normal: A contact normal is a unit vector that points from one shape to another.
접촉 법선은 한 모양에서 다른 모양을 가리키는 단위 벡터입니다.
You can get the PhysicsShape from a contact.
연락처에서 물리적 모양을 얻을 수 있습니다.
From those you can get the bodies.
그들로부터 당신은 물체를 얻을 수 있습니다.
bool onContactBegin(PhysicsContact& contact)
{
auto bodyA = contact.getShapeA()->getBody();
auto bodyB = contact.getShapeB()->getBody();
return true;
}
You can get access to contacts by implementing a contact listener.
연락처 리스너를 구현하여 연락처에 액세스 할 수 있습니다.
The contact listener supports several events: begin, pre-solve, post-solve and separate.
컨택 리스너는 시작, 사전 해결, 사후 해결 및 분리와 같은 여러 이벤트를 지원합니다.
-begin: Two shapes just started touching for the first time this step.
이 단계에서 처음으로 두 개의 모양이 만나기 시작했습니다.
Return true from the callback to process the collision normally or false to cause physics engine to ignore the collision entirely.
콜백에서 true를 반환하여 충돌을 정상적으로 처리하거나 false를 반환하여 물리 엔진이 충돌을 완전히 무시하도록합니다.
If you return false, the preSolve() and postSolve() callbacks will never be run, but you will still receive a separate event when the shapes stop overlapping.
false를 반환하면 pre solve () 및 post solve () 콜백이 실행되지 않지만 셰이프가 겹치지 않으면 별도의 이벤트가 계속 수신됩니다.
-pre-solve: Two shapes are touching during this step.
이 단계에서 두 개의 모양이 접촉합니다.
Return false from the callback to make physics engine ignore the collision this step or true to process it normally.
콜백에서 false를 반환하여 물리 엔진이이 단계에서 충돌을 무시하도록하거나 정상적으로 처리하려면 true를 반환합니다.
Additionally, you may override collision values using setRestitution(), setFriction() or setSurfaceVelocity() to provide custom restitution, friction, or surface velocity values.
추가로, set restitution ()을 사용하여 충돌 값을 재정의하거나, 마찰 ()을 설정하거나, 표면 속도 ()를 설정하여 사용자 지정 복원, 마찰 또는 표면 속도 값을 제공 할 수 있습니다.
-post-solve: Two shapes are touching and their collision response has been processed.
두 모양이 접촉하고 충돌 응답이 처리되었습니다.
-separate: Two shapes have just stopped touching for the first time this step.
이 단계에서 처음으로 두 개의 모양이 터치를 멈췄습니다.
You also can use EventListenerPhysicsContactWithBodies, EventListenerPhysicsContactWithShapes, EventListenerPhysicsContactWithGroup to listen for the event you’re interested with bodies, shapes or groups.
또한 바디와의 이벤트 리스너 물리 접촉, 모양과의 이벤트 리스너 물리 접촉, 그룹과의 이벤트 리스너 물리 접촉을 사용하여 바디, 모양 또는 그룹에 관심이있는 이벤트를 수신 할 수 있습니다.
Besides this you also need to set the physics contact related bitmask value, as the contact event won’t be received by default, even if you create the relative EventListener.
이 외에도 상대 이벤트 리스너를 생성하더라도 기본적으로 접촉 이벤트가 수신되지 않으므로 물리 접촉 관련 비트 마스크 값도 설정해야합니다.
For example:
bool init()
{
auto visibleSize = Director::getInstance()->getVisibleSize();
s_centre = Vec2(visibleSize.width/2, visibleSize.height/2);
//create a static PhysicsBody
// 정적 인 물리 몸체 생성
auto sprite = addSpriteAtPosition(s_centre, 1);
sprite->setTag(10);
sprite->getPhysicsBody()->setContactTestBitmask(0xFFFFFFFF);
sprite->getPhysicsBody()->setDynamic(false);
//adds contact event listener
// 접촉 이벤트 리스너 추가
auto contactListener = EventListenerPhysicsContact::create();
contactListener->onContactBegin = CC_CALLBACK_1(PhysicsDemoCollisionProcessing::onContactBegin, this);
_eventDispatcher->addEventListenerWithSceneGraphPriority(contactListener, this);
schedule(CC_SCHEDULE_SELECTOR(PhysicsDemoCollisionProcessing::tick), 0.3f);
return true;
return false;
}
void tick(float dt)
{
auto sprite1 = addSpriteAtPosition(Vec2(s_centre.x + cocos2d::random(-300,300),
s_centre.y + cocos2d::random(-300,300)));
auto physicsBody = sprite1->getPhysicsBody();
physicsBody->setVelocity(Vec2(cocos2d::random(-500,500),cocos2d::random(-500,500)));
physicsBody->setContactTestBitmask(0xFFFFFFFF);
}
bool onContactBegin(PhysicsContact& contact)
{
auto nodeA = contact.getShapeA()->getBody()->getNode();
auto nodeB = contact.getShapeB()->getBody()->getNode();
if (nodeA && nodeB)
{
if (nodeA->getTag() == 10)
{
nodeB->removeFromParentAndCleanup(true);
}
else if (nodeB->getTag() == 10)
{
nodeA->removeFromParentAndCleanup(true);
}
}
//bodies can collide
// 몸이 충돌 할 수 있음
return true;
}
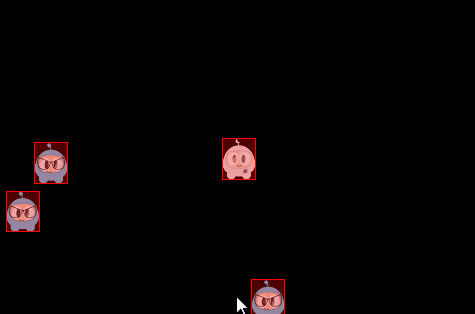